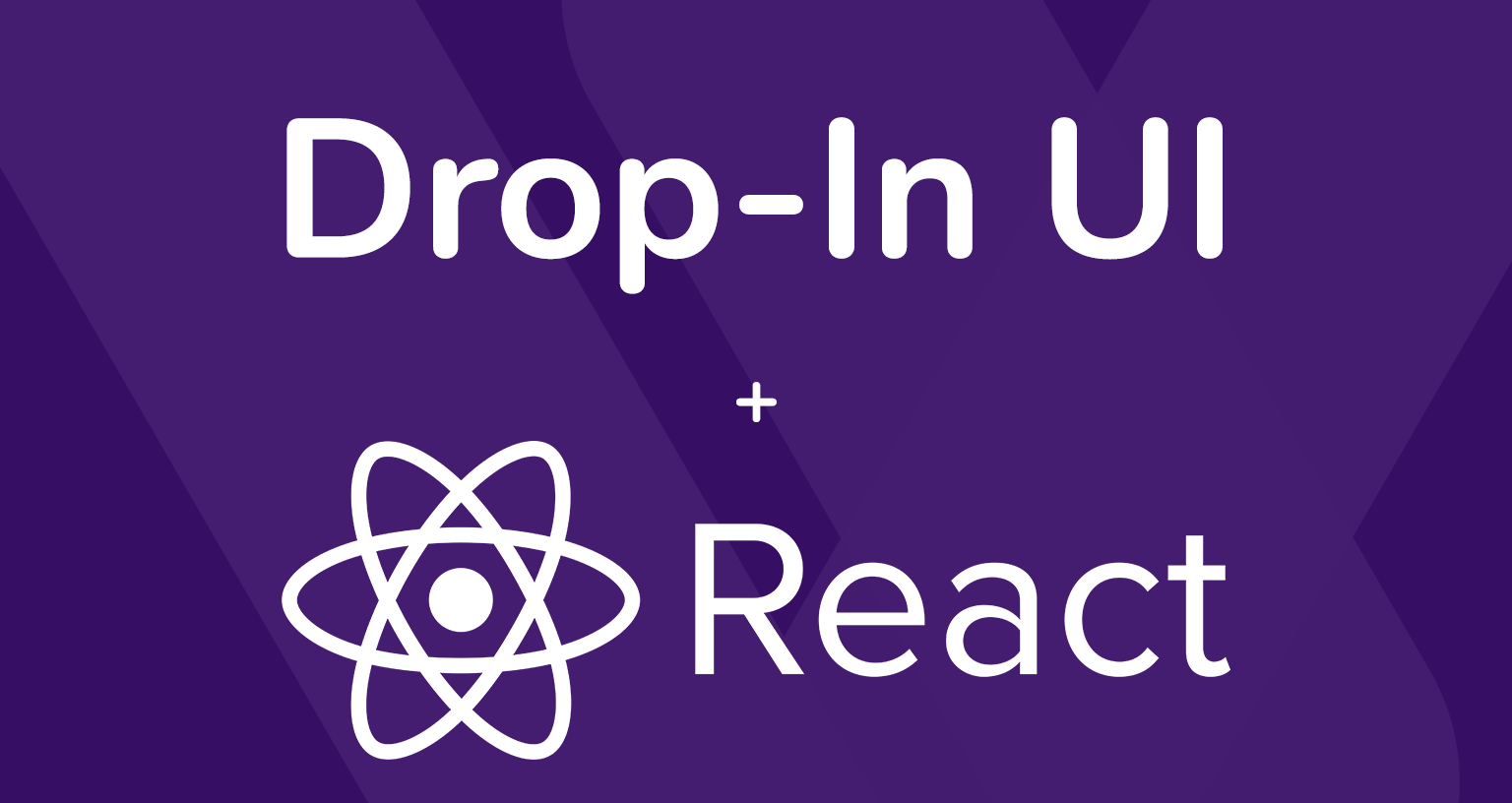
For this tutorial, we are going to be going over how to build a chat app in React by utilizing Weavy's free Chat API & Weavy Cloud. One way to implement Weavy is by utilizing the Drop-in UI and that is the approach we are going to be taking here. By doing so, you can add a full functioning chat to your app within a matter of moments.
**While our React UI Kit is what we recommend for any React projects, the Drop-in UI Kit can come in handy if you have multiple frameworks for your app or one we don't have a specific UI Kit for since it can be utilized with any framework.
For more tutorials, check out Weavy Docs.
Prerequisites
- React 16.8 or higher
- Weavy X or higher
You may also integrate Weavy into an existing app instead of creating a new app, just skip the create a new react app step.
Create a new react app using NPX
NPX is the script manager that comes with Node and NPM, which you may use to create a new react app. React will recommend you to use yarn
or npm
depending on if you have yarn installed or not.
npx create-react-app weavy-react-app
cd weavy-react-app
yarn start
This will build and launch the dev server, usually on http://localhost:3000
Drop-in UI
The Drop-in UI is a javascript library that you add to your existing app or website. The library enables you to quickly add collaboration features such as messaging, document collaboration, and more to your application. It handles Single-Sign-On (SSO) between your app and Weavy, and features an api for initializing and rendering weavy apps that served from the Weavy.Dropin
area on the Weavy backend.
** Please note, we recommend utilizing our React UI Kit for any React projects.
Installation
The easiest way to install the library is by adding it with npm install
, but you can also clone the weavy-dropin-js repo on GitHub.
npm install @weavy/dropin-js
Installing dependencies
The Drop-in UI is also dependent on the theme library that is shared by all our UI kits. If you are using npm, you can install the @weavy/themes
package.
In your project directory, run:
npm install @weavy/themes
Create a shared Weavy context
To be able to link the Weavy instance to Weavy Chat without passing down properties we take advantage of a shared state using a shared context. The Weavy instance component will act as the provider and Weavy Chat component as the consumer. Create a new file src/weavy/WeavyContext.js
to hold the shared context.
WeavyContext.js
import React from 'react';
export default React.createContext();
Create a Weavy instance component
To optimize performance we use a common Weavy instance in a component src/weavy/WeavyWrapper.js
. The instance is linked to the Weavy Chat using the Weavy context. To provide authentication, we prepare the instance to connect a JWT token provider from a property. We delay the initialization to provide recommended creation and cleanup.
The minimum configuration requires the url
to your weavy backend and a jwt
factory function that provides authentication tokens from your server. See authentication for additional details on how to implement this function. Finally, you also need to some required default styling by pointing to the weavy-dropin.css
stylesheet found in the dist
folder of the npm package.
WeavyWrapper.js
import { Component } from 'react';
import './Weavy.css';
import Weavy from "@weavy/dropin-js";
import WeavyContext from './WeavyContext';
export default class WeavyWrapper extends Component {
constructor(props) {
super(props);
this.state = { weavy: window.weavy = new Weavy({
url: "https://localhost:5001",
jwt: () => props.jwt,
})};
};
componentDidMount() {
this.state.weavy.init();
}
componentWillUnmount() {
this.state.weavy.destroy();
};
render() {
return <WeavyContext.Provider value={this.state}>{this.props.children}</WeavyContext.Provider>;
};
}
Create a WeavyChat component
The WeavyChat component src/weavy/WeavyChat.js
will define a Weavy App in a div. The Weavy instance will be referenced from the consumed context. The chat app is defined from component properties.
Apps are used in Weavy to group users around a subject or entity. Within those apps are members. Any members within an app have access to any content and users within that app.
In order to set up the Weavy App, we need a unique identifier - the id. Ids must be unique because they are used to grab the contextual information for that specific space or app. Any unique identifier will do - it just can't be too long.
WeavyChat.js
import { Component } from 'react';
import './Weavy.css';
import WeavyContext from './WeavyContext';
export default class WeavyChat extends Component {
static contextType = WeavyContext;
async createWeavyChat() {
this.weavyChat = this.weavy.app({
id: this.props.appId,
type: this.props.appType,
container: this.el,
open: true,
badge: true
});
}
componentDidMount() {
this.weavy = this.context.weavy;
this.createWeavyChat();
}
shouldComponentUpdate(nextProps){
// A id must change for the app to change
var appChanged = nextProps.appId !== this.props.appId;
return appChanged;
}
componentDidUpdate(prevProps) {
this.weavyChat.remove();
this.createWeavyChat();
}
componentWillUnmount() {
this.weavyChat.remove();
};
render() {
return <div className="weavy-container" ref={el => this.el = el} />;
};
}
Style the Weavy container
Weavy apps will automatically size themselves to the container, so we need to set the size on the Weavy container. Set the height in a stylesheet src/weavy/Weavy.css
Weavy.css
.weavy-container {
height: 100vh;
}
Using the Weavy components
The WeavyWrapper component is only used as a wrapper. You can put it anywhere in your app. The important thing is to place the Weavy Chat component anywhere inside the wrapper. Import the components in your src/App.js
and use the components. You also need to define a JWT token provider and set it as a property on the Weavy instance component.
See Docs - Client Authentication to read more about setting up JWT authentication.
JWT Token must be set up correctly with exp, iss, sub, and the client secret in order for Weavy to work
Both the WeavyWrapper Component and the WeavyChat Component will clean up themselves when removed.
App.js
import './App.css';
import WeavyWrapper from './Weavy/WeavyWrapper';
import WeavyChat from './Weavy/WeavyChat';
function App() {
return (
<WeavyWrapper jwt="eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpc3MiOiJjbGllbnRpZCIsInN1YiI6InN1YjEiLCJuYW1lIjoiRGF2ZSBLb25jc29sIiwiaWF0IjoxNTE2MjM5MDIyLCJleHAiOjIyMzQ1Njc3ODh9.1QLxnyMF0cTKWpcQWy1SsFIHzFb2zs5LYxklpxH0oEw" >
<div className="App">
<WeavyChat
appId="app-id"
appType="messenger"
/>
</div>
</WeavyWrapper>
);
}
export default App;
That's it!
Now you have a WeavyChat component to integrate anywhere in your React web app!
