Note: this tutorial was written for Weavy v8, which uses and different codebase than the current version of Weavy.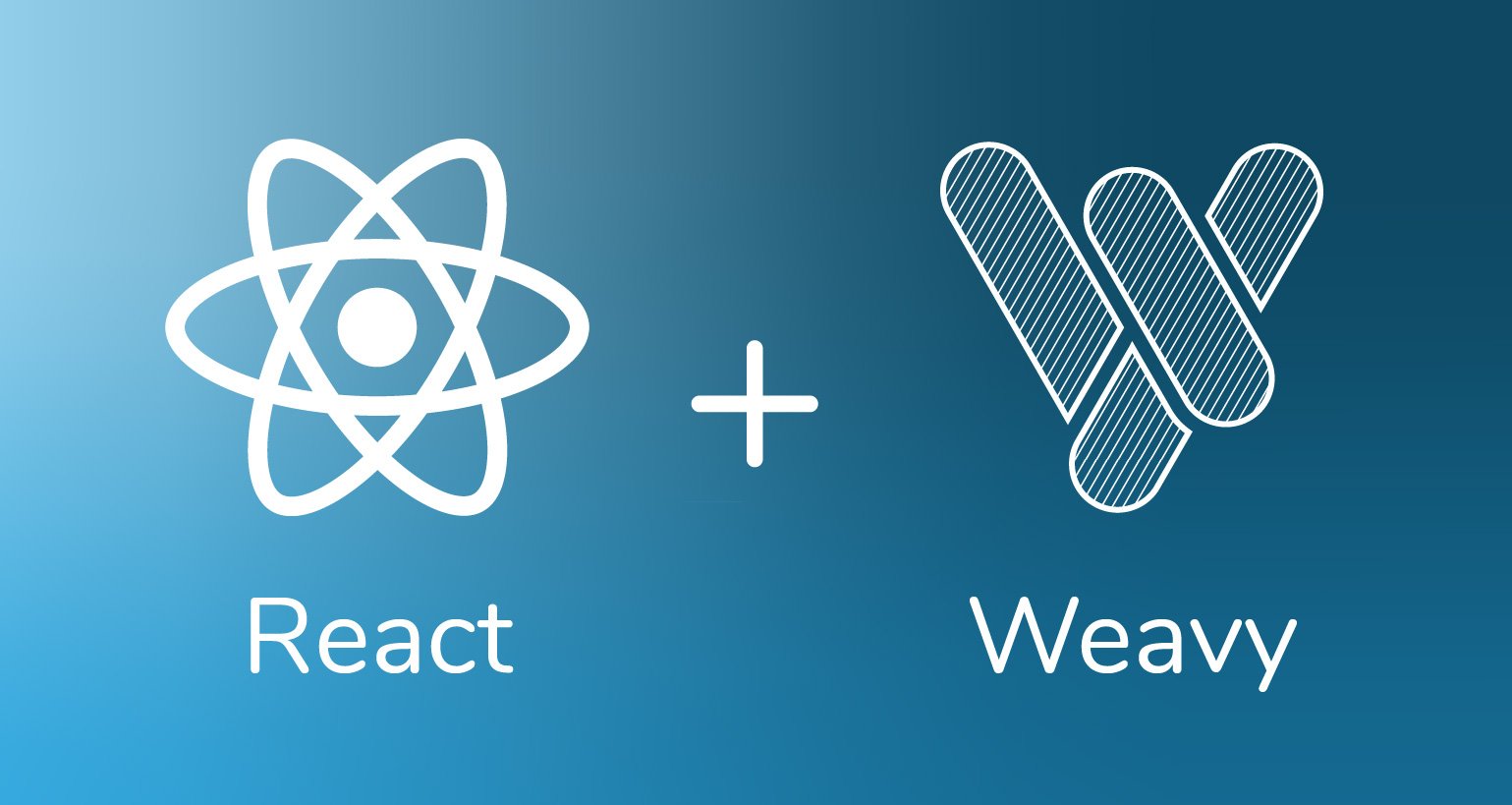
This will guide you to create a basic React web app with Weavy integrated. It will require React 16.8 or higher and Weavy 8.2 or higher. You may also integrate Weavy into an existing app instead of creating a new app, just skip the create a new react app step.
We will create a component for Weavy instances and a component for Weavy apps which will be connected through a shared context.
Create a new react app using NPX
NPX is the script manager that comes with Node and NPM, which you may use to create a new react app. React will recommend you to use yarn
or npm
depending on if you have yarn installed or not.
npx create-react-app weavy-react-app
cd weavy-react-app
yarn start
This will build and launch the dev server, usually on http://localhost:3000
Add the Weavy script
The simplest way to add the Weavy script is to just add it to the head section in the public/index.html
. Replace {your-own-weavy-server}
with the domain name of your own Weavy server.
index.html
<head>
...
<script src="https://code.jquery.com/jquery-3.3.1.min.js"></script>
<script src="https://{your-own-weavy-server}/javascript/weavy.js"></script>
</head>

Create a shared Weavy context
To be able to link the Weavy instance to Weavy apps without passing down properties we take advantage of a shared state using a shared context. The Weavy instance component will act as the provider and Weavy app component as consumer. Create a new file src/weavy/WeavyContext.js
to hold the shared context.
WeavyContext.js
import React from 'react';
export default React.createContext();
Create a Weavy instance component
To optimize performance we use a common Weavy instance in a component src/weavy/Weavy.js
. The instance is linked to the Weavy apps using the Weavy context. To provide authentication we prepare the instance to connect a JWT token provider from a property. We delay the initialization to provide recommended creation and cleanup.
Weavy.js
import { Component } from 'react';
import './Weavy.css';
import WeavyContext from './WeavyContext';
export default class Weavy extends Component {
constructor(props) {
super(props);
this.state = { weavy: new window.Weavy({ jwt: props.jwt, init: false }) };
};
componentDidMount() {
this.state.weavy.init();
}
componentWillUnmount() {
this.state.weavy.destroy();
};
render() {
return <WeavyContext.Provider value={this.state}>{this.props.children}</WeavyContext.Provider>;
};
}
Create a Weavy app component
The Weavy app component src/weavy/WeavyApp.js
will define a Weavy space, then define a Weavy app in a div. The Weavy instance will be references from the consumed context. The space and app is defined from component properties.
WeavyApp.js
import { Component } from 'react';
import './Weavy.css';
import WeavyContext from './WeavyContext'
export default class WeavyApp extends Component {
static contextType = WeavyContext;
async createWeavyApp() {
this.weavySpace = this.weavy.space({
key: this.props.spaceKey,
name: this.props.spaceName
});
this.weavyApp = this.weavySpace.app({
key: this.props.appKey,
type: this.props.appType,
name: this.props.appName,
container: this.el
});
}
componentDidMount() {
this.weavy = this.context.weavy;
this.createWeavyApp();
}
shouldComponentUpdate(nextProps){
// A key must change for the app to change
var spaceChanged = nextProps.spaceKey !== this.props.spaceKey;
var appChanged = nextProps.appKey !== this.props.appKey;
return spaceChanged || appChanged;
}
componentDidUpdate(prevProps) {
this.weavyApp.remove();
this.createWeavyApp();
}
componentWillUnmount() {
this.weavyApp.remove();
};
render() {
return <div className="weavy-container" ref={el => this.el = el} />;
};
}
Style the Weavy container
Weavy apps will automatically size themselves to the container, so we need to set a size on the Weavy container. Set the height in a stylesheet src/weavy/Weavy.css
Weavy.css
.weavy-container {
height: 300px;
}
Using the Weavy components
The Weavy instance component is only used as a wrapper. You can put it anywhere in your app and you may use multiple instances if you like. The important thing is to place the Weavy app components anywhere inside the wrapper. Import the components in your src/App.js
and use the components. You also need to define a JWT token provider and set it as a property on the Weavy instance component.
See docs.weavy.com/client/authentication to read more about setting up JWT authentication.
Both the Weavy Component and the WeavyApp Component will clean up themselves when removed.
App.js
import { Component } from 'react';
import './App.css';
import Weavy from './weavy/Weavy';
import WeavyApp from './weavy/WeavyApp';
export default class App extends Component {
async getJwt() {
return '[Provide your JWT here]';
}
render() {
return (
<Weavy jwt={this.getJwt}>
<div className="App">
<WeavyApp
spaceKey="react-space"
spaceName="React Space"
appKey="react-files"
appName="React Files"
appType="files"
/>
</div>
</Weavy>
);
}
}
Thats it!
Now you have fully customizable Weavy components to integrate anywhere in your web app!
